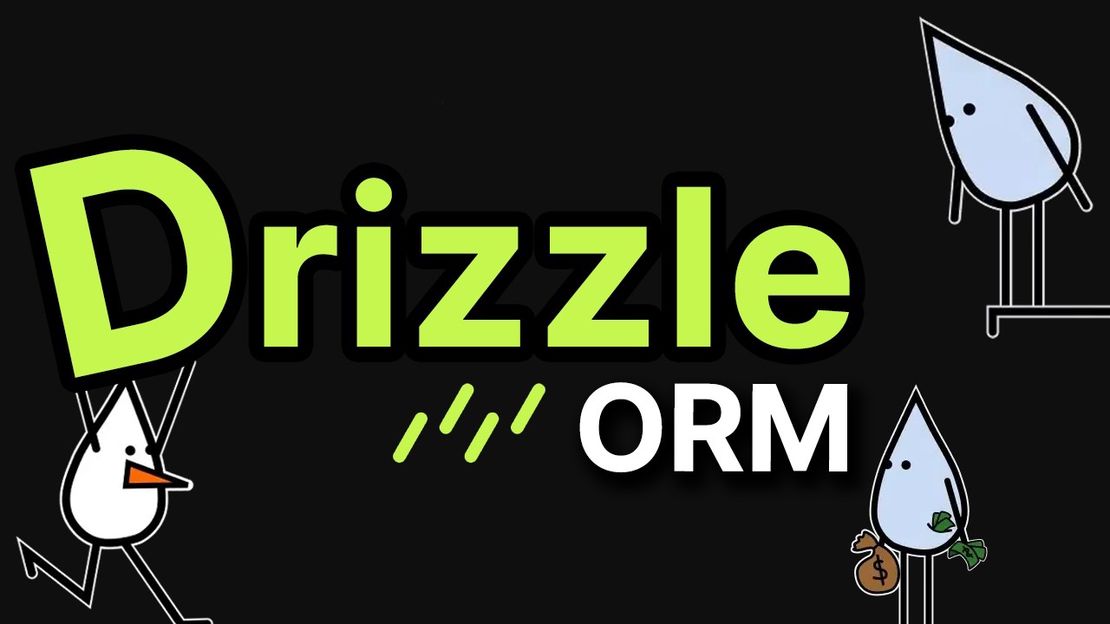
Cascading Deletes in Drizzle
- Atul
- Database , Technology , Programming
- February 18, 2025
Table of Contents
Drizzle
Site URL: https://orm.drizzle.team/
Problem statement:
// Student Table
export const StudentTable = pgTable(
"students",
{
id: uuid("id").defaultRandom().primaryKey().notNull(),
rollNumber: integer("roll_number").notNull(),
enrollmentNumber: text("enrollment_number"),
admissionYear: integer("admission_year").notNull(),
academicYear: text("academic_year"),
currentPhaseId: uuid("current_phase_id")
.notNull()
.references(() => AcademicPhaseTable.id),
studentName: text("student_name"),
studentMobile: text("student_mobile"),
fatherName: text("father_name"),
fatherMobile: text("father_mobile"),
fatherEmail: text("father_email"),
motherName: text("mother_name"),
motherMobile: text("mother_mobile"),
faceDescriptor: json("face_descriptor"),
createdBy: uuid("create_by").references(() => UserTable.id),
createdAt: timestamp("created_at").defaultNow().notNull(),
updatedAt: timestamp("updated_at").notNull(),
},
(table) => ({
rollNumberKey: uniqueIndex(
"students_roll_number_enrollment_admission_key"
).on(table.rollNumber, table.enrollmentNumber, table.admissionYear),
currentPhaseIdx: index("students_current_phase_idx").on(
table.currentPhaseId
),
})
);
export const StudentRelations = relations(StudentTable, ({ one, many }) => ({
currentPhase: one(AcademicPhaseTable, {
fields: [StudentTable.currentPhaseId],
references: [AcademicPhaseTable.id],
}),
phaseHistory: many(StudentPhaseHistoryTable),
attendances: many(AttendanceTable),
batchAssignments: many(StudentSubjectBatchTable),
}));
this is my student table in drizzle
and i want to add a thing like
if i delete a record from this table, other records from other table is deleted
how to do to that?
Here i have used Claude.ai
// Student Table
export const StudentTable = pgTable(
"students",
{
id: uuid("id").defaultRandom().primaryKey().notNull(),
rollNumber: integer("roll_number").notNull(),
enrollmentNumber: text("enrollment_number"),
admissionYear: integer("admission_year").notNull(),
academicYear: text("academic_year"),
currentPhaseId: uuid("current_phase_id")
.notNull()
.references(() => AcademicPhaseTable.id, { onDelete: "cascade" }),
studentName: text("student_name"),
studentMobile: text("student_mobile"),
fatherName: text("father_name"),
fatherMobile: text("father_mobile"),
fatherEmail: text("father_email"),
motherName: text("mother_name"),
motherMobile: text("mother_mobile"),
faceDescriptor: json("face_descriptor"),
createdBy: uuid("create_by").references(() => UserTable.id, { onDelete: "set null" }),
createdAt: timestamp("created_at").defaultNow().notNull(),
updatedAt: timestamp("updated_at").notNull(),
},
(table) => ({
rollNumberKey: uniqueIndex(
"students_roll_number_enrollment_admission_key"
).on(table.rollNumber, table.enrollmentNumber, table.admissionYear),
currentPhaseIdx: index("students_current_phase_idx").on(
table.currentPhaseId
),
})
);
// Student Relations without onDelete (it's handled in the referenced tables)
export const StudentRelations = relations(StudentTable, ({ one, many }) => ({
currentPhase: one(AcademicPhaseTable, {
fields: [StudentTable.currentPhaseId],
references: [AcademicPhaseTable.id],
}),
phaseHistory: many(StudentPhaseHistoryTable),
attendances: many(AttendanceTable),
batchAssignments: many(StudentSubjectBatchTable),
}));
// The cascade delete should be defined in the referenced tables like this:
export const StudentPhaseHistoryTable = pgTable("student_phase_history", {
id: uuid("id").defaultRandom().primaryKey().notNull(),
studentId: uuid("student_id")
.notNull()
.references(() => StudentTable.id, { onDelete: "cascade" }),
// ... other fields
});
export const AttendanceTable = pgTable("attendance", {
id: uuid("id").defaultRandom().primaryKey().notNull(),
studentId: uuid("student_id")
.notNull()
.references(() => StudentTable.id, { onDelete: "cascade" }),
// ... other fields
});
export const StudentSubjectBatchTable = pgTable("student_subject_batch", {
id: uuid("id").defaultRandom().primaryKey().notNull(),
studentId: uuid("student_id")
.notNull()
.references(() => StudentTable.id, { onDelete: "cascade" }),
// ... other fields
});
. In Drizzle, the cascade delete behavior needs to be defined in the referenced table’s foreign key rather than in the relations configuration